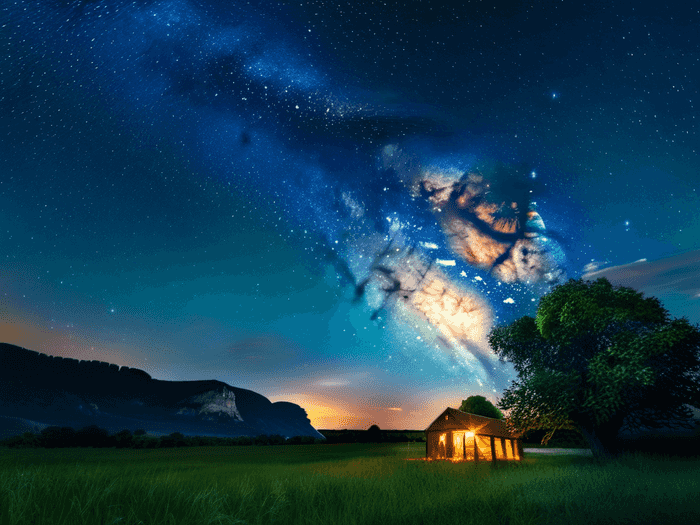
做自己的英雄为您分享以下优质知识
在Javascript中,将星期数字转换为汉字可以通过以下方法实现:
一、使用`getDay()`方法
`Date`对象的`getDay()`方法返回一个0-6的数字,表示星期日到星期六。需要通过数组映射转换为中文汉字。
示例代码:
```javascript
function getChineseWeekday(date) {
const weekdays = ["星期日", "星期一", "星期二", "星期三", "星期四", "星期五", "星期六"];
return weekdays[date.getDay()];
}
// 获取当前日期的星期
const today = new Date();
console.log(getChineseWeekday(today)); // 输出:星期三
```
说明:
1. 定义一个包含中文星期名称的数组`weekdays`。
2. 使用`date.getDay()`获取星期数字(0-6)。
3. 通过数组索引直接获取对应的中文星期名称。
二、格式化日期为"星期X"字符串
如果需要将日期与时间组合成"星期X, 日期"的格式,可以扩展上述函数:
```javascript
function formatDateWithWeekday(date) {
const weekdays = ["星期日", "星期一", "星期二", "星期三", "星期四", "星期五", "星期六"];
const day = weekdays[date.getDay()];
const year = date.getFullYear();
const month = String(date.getMonth() + 1).padStart(2, '0'); // 月份从0开始,需加1
const dayOfMonth = String(date.getDate()).padStart(2, '0');
return `${day}, ${year}-${month}-${dayOfMonth}`;
}
// 示例
console.log(formatDateWithWeekday(new Date())); // 输出:星期三, 2025-04-16
```
三、处理多天日期范围
若需展示连续多天的星期名称(如"周一至周五"),可遍历日期数组并拼接:
```javascript
function getWeekRange(startDate, endDate) {
const weekdays = ["星期日", "星期一", "星期二", "星期三", "星期四", "星期五", "星期六"];
const range = [];
let currentDate = new Date(startDate);
while (currentDate